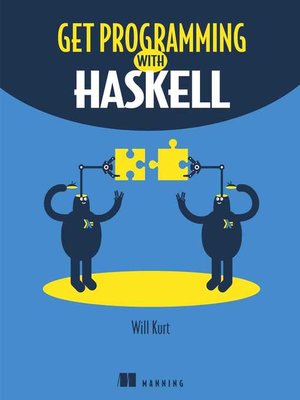
Sign up to save your library
With an OverDrive account, you can save your favorite libraries for at-a-glance information about availability. Find out more about OverDrive accounts.
Find this title in Libby, the library reading app by OverDrive.
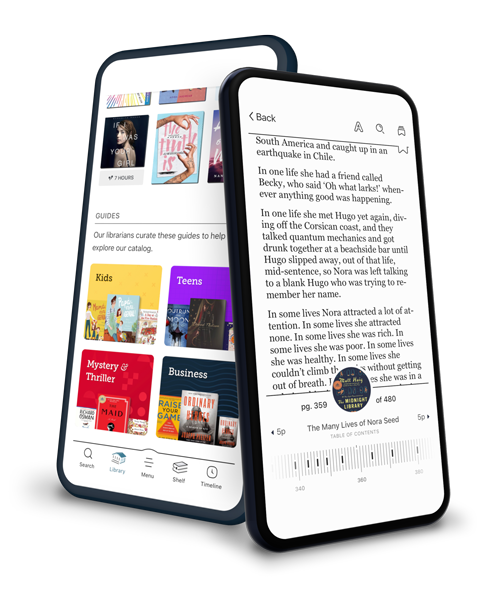
Search for a digital library with this title
Title found at these libraries:
Library Name | Distance |
---|---|
Loading... |
Summary
Get Programming with Haskell leads you through short lessons, examples, and exercises designed to make Haskell your own. It has crystal-clear illustrations and guided practice. You will write and test dozens of interesting programs and dive into custom Haskell modules. You will gain a new perspective on programming plus the practical ability to use Haskell in the everyday world. (The 80 IQ points: not guaranteed.)
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Programming languages often differ only around the edges—a few keywords, libraries, or platform choices. Haskell gives you an entirely new point of view. To the software pioneer Alan Kay, a change in perspective can be worth 80 IQ points and Haskellers agree on the dramatic benefits of thinking the Haskell way—thinking functionally, with type safety, mathematical certainty, and more. In this hands-on book, that's exactly what you'll learn to do.
What's Inside
Thinking in Haskell Functional programming basics Programming in types Real-world applications for Haskell
About the Reader
Written for readers who know one or more programming languages.
Table of Contents
Lesson 1 Getting started with Haskell
Unit 1 - FOUNDATIONS OF FUNCTIONAL PROGRAMMING
Lesson 2 Functions and functional programming
Lesson 3 Lambda functions and lexical scope
Lesson 4 First-class functions
Lesson 5 Closures and partial application
Lesson 6 Lists
Lesson 7 Rules for recursion and pattern matching
Lesson 8 Writing recursive functions
Lesson 9 Higher-order functions
Lesson 10 Capstone: Functional object-oriented programming with robots!
Unit 2 - INTRODUCING TYPES
Lesson 11 Type basics
Lesson 12 Creating your own types
Lesson 13 Type classes
Lesson 14 Using type classes
Lesson 15 Capstone: Secret messages!
Unit 3 - PROGRAMMING IN TYPES
Lesson 16 Creating types with "and" and "or"
Lesson 17 Design by composition—Semigroups and Monoids
Lesson 18 Parameterized types
Lesson 19 The Maybe type: dealing with missing values
Lesson 20 Capstone: Time series
Unit 4 - IO IN HASKELL
Lesson 21 Hello World!—introducing IO types
Lesson 22 Interacting with the command line and lazy I/O
Lesson 23 Working with text and Unicode
Lesson 24 Working with files
Lesson 25 Working with binary data
Lesson 26 Capstone: Processing binary files and book data
Unit 5 - WORKING WITH TYPE IN A CONTEXT
Lesson 27 The Functor type class
Lesson 28 A peek at the Applicative type class: using functions in a context
Lesson 29 Lists as context: a deeper look at the Applicative type class
Lesson 30 Introducing the Monad type class
Lesson 31 Making Monads easier with donotation
Lesson 32 The list monad and list comprehensions
Lesson 33 Capstone: SQL-like queries in Haskell
Unit 6 - ORGANIZING CODE AND BUILDING PROJECTS
Lesson 34 Organizing Haskell code with modules
Lesson 35 Building projects with stack
Lesson 36 Property testing with QuickCheck
Lesson 37 Capstone: Building a prime-number library
Unit 7 - PRACTICAL HASKELL
Lesson 38 Errors in Haskell and the Either type
Lesson 39 Making HTTP requests in Haskell
Lesson 40 Working with JSON data by using Aeson
Lesson 41 Using databases in Haskell
Lesson 42 Efficient, stateful arrays in Haskell
Afterword - What's next?
Appendix - Sample answers to exercise
Get Programming with Haskell leads you through short lessons, examples, and exercises designed to make Haskell your own. It has crystal-clear illustrations and guided practice. You will write and test dozens of interesting programs and dive into custom Haskell modules. You will gain a new perspective on programming plus the practical ability to use Haskell in the everyday world. (The 80 IQ points: not guaranteed.)
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Programming languages often differ only around the edges—a few keywords, libraries, or platform choices. Haskell gives you an entirely new point of view. To the software pioneer Alan Kay, a change in perspective can be worth 80 IQ points and Haskellers agree on the dramatic benefits of thinking the Haskell way—thinking functionally, with type safety, mathematical certainty, and more. In this hands-on book, that's exactly what you'll learn to do.
What's Inside
About the Reader
Written for readers who know one or more programming languages.
Table of Contents
Lesson 1 Getting started with Haskell
Unit 1 - FOUNDATIONS OF FUNCTIONAL PROGRAMMING
Lesson 2 Functions and functional programming
Lesson 3 Lambda functions and lexical scope
Lesson 4 First-class functions
Lesson 5 Closures and partial application
Lesson 6 Lists
Lesson 7 Rules for recursion and pattern matching
Lesson 8 Writing recursive functions
Lesson 9 Higher-order functions
Lesson 10 Capstone: Functional object-oriented programming with robots!
Unit 2 - INTRODUCING TYPES
Lesson 11 Type basics
Lesson 12 Creating your own types
Lesson 13 Type classes
Lesson 14 Using type classes
Lesson 15 Capstone: Secret messages!
Unit 3 - PROGRAMMING IN TYPES
Lesson 16 Creating types with "and" and "or"
Lesson 17 Design by composition—Semigroups and Monoids
Lesson 18 Parameterized types
Lesson 19 The Maybe type: dealing with missing values
Lesson 20 Capstone: Time series
Unit 4 - IO IN HASKELL
Lesson 21 Hello World!—introducing IO types
Lesson 22 Interacting with the command line and lazy I/O
Lesson 23 Working with text and Unicode
Lesson 24 Working with files
Lesson 25 Working with binary data
Lesson 26 Capstone: Processing binary files and book data
Unit 5 - WORKING WITH TYPE IN A CONTEXT
Lesson 27 The Functor type class
Lesson 28 A peek at the Applicative type class: using functions in a context
Lesson 29 Lists as context: a deeper look at the Applicative type class
Lesson 30 Introducing the Monad type class
Lesson 31 Making Monads easier with donotation
Lesson 32 The list monad and list comprehensions
Lesson 33 Capstone: SQL-like queries in Haskell
Unit 6 - ORGANIZING CODE AND BUILDING PROJECTS
Lesson 34 Organizing Haskell code with modules
Lesson 35 Building projects with stack
Lesson 36 Property testing with QuickCheck
Lesson 37 Capstone: Building a prime-number library
Unit 7 - PRACTICAL HASKELL
Lesson 38 Errors in Haskell and the Either type
Lesson 39 Making HTTP requests in Haskell
Lesson 40 Working with JSON data by using Aeson
Lesson 41 Using databases in Haskell
Lesson 42 Efficient, stateful arrays in Haskell
Afterword - What's next?
Appendix - Sample answers to exercise